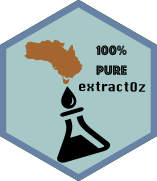
Extract Weather From the Nearest Patched Point Weather Station in the SILO Network Using Australian GPS Coordinates
Source:R/extract_patched_point.R
extract_patched_point.Rd
Extracts station-based weather data for the provided dates from the nearest
weather station to the provided longitude and latitude values in x
.
Weather data for the single closest station(s) to the provided longitude
and latitude will always be returned.
Usage
extract_patched_point(
x,
start_date,
end_date,
n_stations = 1L,
values = "all",
api_key = NULL
)
Arguments
- x
List
. An object with names containingvector
pairings of longitude and latitude values expressed as decimal degree values in that order, each individualvectors
' items should be named "x" (longitude) and "y" (latitude), respectively. Thelist
item names should be descriptive of the individualvectors
and will be included in a "location" column of the output.- start_date
A
character
string orDate
object representing the beginning of the range to query in the format “yyyy-mm-dd” (ISO8601). Data returned is inclusive of this date.- end_date
A
character
string orDate
object representing the end of the range query in the format “yyyy-mm-dd” (ISO8601). Data returned is inclusive of this date. Defaults to the current system date.- n_stations
An
integer
value that indicates how many stations per location should be returned. Defaults to one (1) station with only the nearest station being returned. Values greater than 1 will result in the next nearest stations being returned from 1 to n.- values
A
character
string with the type of weather data to return. See Available Values for a full list of valid values. Defaults toall
with all available values being returned.- api_key
A
character
string specifying a valid email address to use for the request. The query will return an error if a valid email address is not provided.
Value
a data.table::data.table with the weather data queried with the weather variables in alphabetical order. The first eight columns will always be:
station_code
,station_name
,longitude
,latitude
,elev_m
(elevation in metres),date
(ISO8601 format, "YYYYMMDD"),year
,month
,day
,extracted
(the date on which the query was made)
Column Name Details
Column names are converted from the default returns of the API to be
snake_case formatted and where appropriate, the names of the values that
are analogous between SILO and DPIRD data are named
using the same name for ease of interoperability, e.g., using
rbind()
to create a data.table
that contains data from both APIs.
The SILO documentation provides the following information for the PatchedPoint data.
These data are a continuous daily time series of data at either recording stations or grid points across Australia:
Data at station locations consists of observational records which have been supplemented by interpolated estimates when observed data are missing. Datasets are available at approximately 8,000 Bureau of Meteorology recording stations around Australia.
Data at grid points consists entirely of interpolated estimates. The data are taken from the SILO gridded datasets and are available at any pixel on a 0.05° × 0.05° grid over the land area of Australia (including some islands).
Available Values
- all
Which will return all of the following values
- rain (mm)
Rainfall
- max_temp (degrees C)
Maximum temperature
- min_temp (degrees C)
Minimum temperature
- vp (hPa)
Vapour pressure
- vp_deficit (hPa)
Vapour pressure deficit
- evap_pan (mm)
Class A pan evaporation
- evap_syn (mm)
Synthetic estimate1
- evap_comb (mm)
Combination (synthetic estimate pre-1970, class A pan 1970 onwards)
- evap_morton_lake (mm)
Morton's shallow lake evaporation
- radiation (Mj/m2)
Solar exposure, consisting of both direct and diffuse components
- rh_tmax (%)
Relative humidity at the time of maximum temperature
- rh_tmin (%)
Relative humidity at the time of minimum temperature
- et_short_crop (mm)
FAO564 short crop
- et_tall_crop (mm)
ASCE5 tall crop6
- et_morton_actual (mm)
Morton's areal actual evapotranspiration
- et_morton_potential (mm)
Morton's point potential evapotranspiration
- et_morton_wet (mm)
Morton's wet-environment areal potential evapotranspiration over land
- mslp (hPa)
Mean sea level pressure
Value information
Solar radiation: total incoming downward shortwave radiation on a horizontal surface, derived from estimates of cloud oktas and sunshine duration3.
Relative humidity: calculated using the vapour pressure measured at 9am, and the saturation vapour pressure computed using either the maximum or minimum temperature6.
Evaporation and evapotranspiration: an overview of the variables provided by SILO is available here, https://data.longpaddock.qld.gov.au/static/publications/Evapotranspiration_overview.pdf.
Data codes
The data are supplied with codes indicating how each datum was obtained.
- 0
Official observation as supplied by the Bureau of Meteorology
- 15
Deaccumulated rainfall (original observation was recorded over a period exceeding the standard 24 hour observation period).
- 25
Interpolated from daily observations for that date.
- 26
Synthetic Class A pan evaporation, calculated from temperatures, radiation and vapour pressure.
- 35
Interpolated from daily observations using an anomaly interpolation method.
- 75
Interpolated from the long term averages of daily observations for that day of year.
References
Rayner, D. (2005). Australian synthetic daily Class A pan evaporation. Technical Report December 2005, Queensland Department of Natural Resources and Mines, Indooroopilly, Qld., Australia, 40 pp.
Morton, F. I. (1983). Operational estimates of areal evapotranspiration and their significance to the science and practice of hydrology, Journal of Hydrology, Volume 66, 1-76.
Zajaczkowski, J., Wong, K., & Carter, J. (2013). Improved historical solar radiation gridded data for Australia, Environmental Modelling & Software, Volume 49, 64–77. DOI: doi:10.1016/j.envsoft.2013.06.013 .
Food and Agriculture Organization of the United Nations, Irrigation and drainage paper 56: Crop evapotranspiration - Guidelines for computing crop water requirements, 1998.
ASCE’s Standardized Reference Evapotranspiration Equation, proceedings of the National Irrigation Symposium, Phoenix, Arizona, 2000.
For further details refer to Jeffrey, S.J., Carter, J.O., Moodie, K.B. and Beswick, A.R. (2001). Using spatial interpolation to construct a comprehensive archive of Australian climate data, Environmental Modelling and Software, Volume 16/4, 309-330. DOI: doi:10.1016/S1364-8152(01)00008-1 .
See also
Other weather data:
extract_data_drill()
,
extract_power()
Other SILO:
extract_data_drill()
Author
Adam H. Sparks, adamhsparks@gmail.com
Examples
if (FALSE) { # \dontrun{
# Source data from a list of latitude and longitude coordinates in NSW & WA
locs <- list(
"Merredin" = c(x = 118.28, y = -31.48),
"Corrigin" = c(x = 117.87, y = -32.33),
"Tamworth" = c(x = 150.84, y = -31.07)
)
# Replace "your_api_key" with a valid email address.
w <- extract_patched_point(
x = locs,
start_date = "20210101",
end_date = "20210131",
api_key = "your_api_key"
)
} # }